Maximizing Efficiency in Vue.js: Harnessing the Power of Reusable Components
Vue.js is an open-source JavaScript framework highly sought after by organizations looking to hire Vue.js developers for building efficient user interfaces. A core feature of Vue.js, and a key skill for any Vue.js developers you might hire, is its system of components. In this article, we will explore Vue.js components in detail, focusing on their reusability, a critical aspect when you hire Vue.js developers, as this can greatly enhance your web development process. By understanding these components and their versatile applications, you can set a clear benchmark for the capabilities you expect when you hire Vue.js developers.
1. What are Vue.js Components?
Vue.js components are reusable instances with a name. They are essentially custom elements that Vue’s compiler would attach behavior to. In other words, Vue.js components are chunks of encapsulated code that can be reused across our application. This makes components one of the fundamental building blocks of a Vue.js application, providing a structured and maintainable approach to developing complex user interfaces.
2. Vue.js Components and Reusability
The power of Vue.js components comes from their reusability. By creating a well-structured component, you can reuse it across your application, which leads to less code duplication, more manageable code, and a more consistent user experience.
2.1. Simple Example of a Vue.js Component
To understand how Vue.js components work, let’s start with a simple example. Suppose we want to create a reusable button with a custom label. Here’s how we can do it with Vue.js:
```javascript Vue.component('my-button', { props: ['label'], template: '<button>{{ label }}</button>' }) new Vue({ el: '#app' }) ```
Now, in our HTML, we can use the `my-button` component like this:
```html <div id="app"> <my-button label="Click me"></my-button> </div> ```
2.2. Advancing with Dynamic Components
Dynamic components take reusability a notch higher. Vue provides a special `<component>` element with the `is` attribute that dynamically switches between components, based on the data passed to it. Here’s an example:
```javascript Vue.component('home', { template: '<div>Home</div>' }) Vue.component('about', { template: '<div>About</div>' }) new Vue({ el: '#app', data: { currentTab: 'home' } }) ```
In the HTML, we can use the dynamic component like this:
```html <div id="app"> <component v-bind:is="currentTab"></component> <button v-on:click="currentTab = 'home'">Home</button> <button v-on:click="currentTab = 'about'">About</button> </div> ```
2.3. Slots and Reusability
Slots are another way Vue.js enhances reusability. They allow you to compose components in a way that makes them even more reusable and adaptable to different contexts.
Let’s consider an example of a simple ‘Card’ component:
```javascript Vue.component('card', { template: ` <div class="card"> <div class="card-header"> <slot name="header"></slot> </div> <div class="card-body"> <slot></slot> </div> </div> ` }) ```
This card component has two slots – one unnamed slot for the body content, and a named slot for the header content. It can be reused in different contexts as shown below:
```html <card> <template v-slot:header> <h1>Welcome!</h1> </template> <p>This is some example content for the card body.</p> </card> <card> <template v-slot:header> <h2>About Us</h2> </template> <p>This is some information about us.</p> </ card> ```
3. Composing Components
Composing components is a process of assembling multiple components together to create complex user interfaces. By properly dividing the application’s features into well-encapsulated components, we ensure high reusability and maintainability.
Consider an example of a ‘BlogPost’ component which can be composed from smaller components:
```javascript Vue.component('blog-post', { props: ['post'], template: ` <div class="blog-post"> <h2>{{ post.title }}</h2> <p>{{ post.content }}</p> <comment-list v-bind:comments="post.comments"></comment-list> </div> ` }) Vue.component('comment-list', { props: ['comments'], template: ` <div class="comment-list"> <comment v-for="comment in comments" :comment="comment"></comment> </div> ` }) Vue.component('comment', { props: ['comment'], template: ` <div class="comment"> <div class="comment-author">{{ comment.author }}</div> <div class="comment-body">{{ comment.body }}</div> </div> ` }) ```
In this example, we have a `BlogPost` component, which is composed of a `CommentList` component, which in turn consists of `Comment` components.
4. Advanced Reusability with Mixins
In some scenarios, particularly in complex projects that require you to hire Vue.js developers, using components may not be enough to ensure DRY (Don’t Repeat Yourself) code. Vue.js provides an advanced feature known as mixins for these scenarios. Mixins are a flexible way to distribute reusable functionalities for Vue components. This versatility and sophistication are why many businesses opt to hire Vue.js developers; their skill in employing such features allows for creating highly maintainable and reusable code, contributing to efficient and robust applications.
Suppose you have a `UserGreeting` and `AdminGreeting` components and you want to show a welcome message in both. This can be achieved with a mixin as shown below:
```javascript var GreetingMixin = { created: function () { this.greet() }, methods: { greet: function () { var date = new Date() if (date.getHours() < 12) { alert('Good Morning!') } else if (date.getHours() < 18) { alert('Good Afternoon!') } else { alert('Good Evening!') } } } } var UserGreeting = Vue.component('user-greeting', { mixins: [GreetingMixin] }) var AdminGreeting = Vue.component('admin-greeting', { mixins: [GreetingMixin] }) ```
Conclusion
Vue.js components are a powerful tool that skilled Vue.js developers leverage for creating complex, maintainable, and scalable applications. By taking advantage of the reusable nature of components and various features of Vue.js, these professionals can create clean and DRY code. From simple components to dynamic components, slots, component composition, and mixins, Vue.js offers a range of tools for crafting high-quality web applications. These features make Vue.js one of the most favored frameworks among developers, particularly those looking to hire Vue.js developers, for building efficient and reusable user interfaces.
Remember, the reusability of components is not just about writing less code. It’s about improving maintainability, readability, and scalability, all of which are crucial in a successful, large-scale application. When used correctly, Vue.js components can make a substantial difference in your web development journey. Thus, hiring Vue.js developers with a strong grasp of these concepts can be a strategic move for any project.
Table of Contents
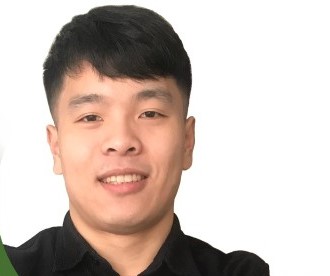
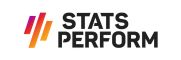