Building a Single-Page Application with Vue.js and Vue Router
Single-page applications (SPAs) have become increasingly popular due to their seamless user experience and efficient use of resources. Vue.js, a progressive JavaScript framework, has emerged as a go-to choice for building SPAs due to its simplicity, flexibility, and high performance. With Vue Router, a powerful routing library for Vue.js, creating SPA navigation becomes a breeze.
Table of Contents
In this comprehensive guide, we will walk you through the process of building a Single-Page Application with Vue.js and Vue Router. By the end of this tutorial, you’ll have a solid foundation in creating dynamic, responsive, and feature-rich web applications.
1. Prerequisites
Before we dive into building our SPA, make sure you have the following prerequisites in place:
- Basic understanding of HTML, CSS, and JavaScript.
- Node.js and npm installed on your system.
2. Setting Up the Project
Let’s start by setting up the project directory and installing the necessary dependencies.
Step 1: Initializing a Vue.js Project
To create a new Vue.js project, open your terminal and run the following command:
bash npm install -g @vue/cli # Install Vue CLI globally (if not already installed) vue create my-spa # Create a new Vue project named 'my-spa' cd my-spa # Navigate to the project directory
During the setup process, Vue CLI will prompt you to choose various project configurations. Feel free to select the options that best suit your project needs.
Step 2: Installing Vue Router
Once the project is initialized, navigate to the project directory in your terminal and install Vue Router:
bash vue add router
This command will add the necessary Vue Router files and configurations to your project.
3. Understanding Vue Router
Before we start building the SPA, let’s briefly understand Vue Router and its key concepts.
3.1. What is Vue Router?
Vue Router is the official routing library for Vue.js. It allows us to create complex navigation within a Vue application by mapping URLs to specific components. When a user navigates to a particular URL, the corresponding component is rendered, providing a seamless SPA experience without full-page reloads.
3.2. Key Concepts
3.2.1. Routes
Routes define the URL paths and the components associated with them. Each route is mapped to a specific component that will be displayed when the corresponding URL is accessed.
3.2.2. Router Instance
The router instance is responsible for creating and managing the routes. It is added to the main Vue instance and allows components to access routing functionality.
3.2.3. Router View
The <router-view> component acts as a placeholder in the template where the matched route’s component will be rendered. It dynamically displays the appropriate component based on the current URL.
3.2.4. Router Link
The <router-link> component is used to navigate between different routes within the SPA. It automatically generates links based on the routes defined in the router instance.
4. Creating the SPA Layout
Now that we have Vue Router set up in our project, let’s create the basic layout for our SPA.
Step 3: Modify App.vue
Open the src/App.vue file in your code editor and update its content as follows:
html <template> <div id="app"> <header> <router-link to="/">Home</router-link> <router-link to="/about">About</router-link> </header> <main> <router-view></router-view> </main> <footer> <!-- Your footer content here --> </footer> </div> </template> <script> export default { name: "App", }; </script> <style> /* Your CSS styles here */ </style>
In the code above, we added a header, main section, and footer to the App.vue file. The <router-link> components are used to navigate between the Home and About pages.
5. Defining Routes
With the layout in place, let’s define the routes for our SPA.
Step 4: Modify router/index.js
Open the src/router/index.js file and define the routes as shown below:
js import Vue from "vue"; import VueRouter from "vue-router"; Vue.use(VueRouter); const routes = [ { path: "/", name: "Home", component: () => import("../views/Home.vue"), }, { path: "/about", name: "About", component: () => import("../views/About.vue"), }, ]; const router = new VueRouter({ routes, }); export default router;
In the code above, we imported Vue Router and set up the routes. We associated the Home route with the Home.vue component and the About route with the About.vue component.
6. Creating the Views
Now that our routes are defined, let’s create the views for the Home and About pages.
Step 5: Create Home.vue and About.vue
In the src/views folder, create two new files: Home.vue and About.vue.
html <!-- Home.vue --> <template> <div class="home"> <h1>Welcome to our SPA</h1> <!-- Add your content here --> </div> </template> <script> export default { name: "Home", }; </script> <style> /* Your CSS styles here */ </style> html <!-- About.vue --> <template> <div class="about"> <h1>About Us</h1> <!-- Add your content here --> </div> </template> <script> export default { name: "About", }; </script> <style> /* Your CSS styles here */ </style>
In the code above, we created basic templates for the Home and About views. Customize them with your content and styles to make them visually appealing.
7. Testing the SPA
With everything set up, it’s time to test our SPA and see it in action.
Step 6: Run the Development Server
In your terminal, navigate to the project directory and start the development server:
bash npm run serve
Once the server is running, visit http://localhost:8080 in your web browser. You should see the home page of your SPA.
Step 7: Navigate to the About Page
Click on the “About” link in the header. The SPA should seamlessly navigate to the About page without a full page reload.
Congratulations! You’ve successfully built a Single-Page Application with Vue.js and Vue Router. You now have a solid foundation to create more complex SPAs with additional features and functionality.
Conclusion
In this blog post, we explored how to build a Single-Page Application using Vue.js and Vue Router. We started by setting up the project and installing Vue Router. Then, we delved into Vue Router’s key concepts, including routes, the router instance, <router-view>, and <router-link>.
Next, we created the basic layout for our SPA and defined routes for the Home and About pages. We also created the corresponding views for these pages to display dynamic content.
Vue.js and Vue Router provide a powerful combination for creating modern, interactive, and user-friendly SPAs. As you continue your journey with Vue.js, you’ll find a wealth of additional features and plugins to enhance your applications further.
Feel free to explore more advanced topics, such as route guards, nested routes, and lazy loading, to take your Vue.js SPA development to the next level. Happy coding!
Table of Contents
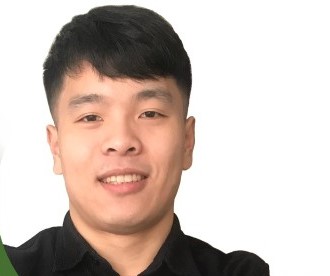
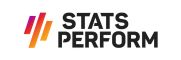