Mastering Interactivity in Vue.js: A Deep Dive into Directives
In modern frontend development, state management is a crucial aspect of any application, and this is no different in Vue.js, which is why many companies decide to hire Vue.js developers for their projects. With Vue.js, Vuex is the most common tool for state management that Vue.js developers utilize. But it’s not the only option – many other libraries and methodologies, known to experienced Vue.js developers, provide similar functionalities, sometimes with different approaches. This blog post aims to illustrate state management in Vue.js using Vuex and highlight some alternatives beyond it, providing useful insights whether you’re planning to hire Vue.js developers or are looking to deepen your own Vue.js knowledge.
What is State Management?
Before we delve into the specifics, it’s important to understand the concept of ‘state’. In frontend development, the state refers to the data that determines the behavior and rendering of your application. State management, then, is about handling this data: how to store, manipulate, and pass it around your application.
The need for effective state management becomes evident as your application grows. Large applications might have numerous components that need to access or modify the same data. Without effective state management, you’ll quickly end up in a maze of data props and event emitters, leading to a codebase that’s hard to understand and maintain.
Vuex – Vue.js Official State Management Library
VuVuex is the most used state management solution for Vue.js applications. It serves as a centralized store for all the components in an application, with rules ensuring that the state can only be mutated predictably. Let’s look at the main elements of Vuex: State, Getters, Mutations, and Actions.
State
The state holds the raw data for your application. Each application has a single state tree. Let’s take an example of a simple blog application:
```js const store = new Vuex.Store({ state: { posts: [] } }) ```
Getters
Getters are the computed properties of the store. You can use them to derive data from the state:
```js const store = new Vuex.Store({ state: { posts: [] }, getters: { postCount: state => state.posts.length } }) ```
Mutations
Mutations are the only way to change state in the store. They are similar to events and are committed to change the state:
```js const store = new Vuex.Store({ state: { posts: [] }, mutations: { addPost: (state, post) => state.posts.push(post) } }) ```
Actions
Actions are similar to mutations, but they commit mutations and can contain arbitrary asynchronous operations:
```js const store = new Vuex.Store({ state: { posts: [] }, mutations: { addPost: (state, post) => state.posts.push(post) }, actions: { addPost: ({ commit }, post) => commit('addPost', post) } }) ```
In a Vue component, you can dispatch an action to add a new post like so:
```js this.$store.dispatch('addPost', newPost) ```
While Vuex is a powerful tool, it might not always be the best fit, especially for small projects or applications with a flat state structure. It also introduces a fair amount of boilerplate code. Let’s look at some alternatives beyond Vuex.
Vue.Observable
Vue.Observable is a simpler, lightweight alternative to Vuex. It uses the Vue reactivity system to create an observable object:
```js const state = Vue.observable({ posts: [] }) const addPost = post => state.posts.push(post) ```
In your components, you can directly access the state or use it in computed properties:
```js computed: { posts: () => state.posts, postCount: () => state.posts.length } ```
While Vue.Observable is less powerful and doesn’t have built-in dev
tools like Vuex, it’s an excellent choice for simple applications or to avoid the boilerplate associated with Vuex.
Pinia
Pinia is a lightweight, intuitive state management library that was created as an alternative to Vuex. It offers a similar API but reduces the amount of boilerplate and improves type inference when used with TypeScript:
```js import { defineStore } from 'pinia' const usePostStore = defineStore({ id: 'posts', state: () => ({ posts: [] }), actions: { addPost(post) { this.posts.push(post) } } }) // to use the store import { usePostStore } from '@/stores/posts' const postStore = usePostStore() postStore.addPost(newPost) ```
Vue Apollo
If your Vue.js application interacts with a GraphQL API, Vue Apollo offers a way to handle state management. With Apollo Client’s caching, you can manage local and remote data efficiently.
```js const POSTS_QUERY = gql` query Posts { posts { id title content } } ` export default { apollo: { posts: { query: POSTS_QUERY, update: data => data.posts } } } ```
Vue Composition API
The Vue Composition API is a new addition in Vue 3, a feature that has made the task of those who hire Vue.js developers even more fruitful. It allows better logic encapsulation and code reuse. While it doesn’t provide a full state management solution by itself, it can be used with the Vue reactivity system to manage state efficiently. This innovation is part of what makes Vue.js developers in high demand, as they can leverage such powerful tools for optimal frontend development.
```js import { reactive, toRefs } from 'vue' const state = reactive({ posts: [] }) export function usePosts() { const addPost = post => state.posts.push(post) return { ...toRefs(state), addPost } } ```
In your components, you can use the `usePosts` function to access and modify the posts:
```js import { usePosts } from '@/composables/usePosts' export default { setup() { const { posts, addPost } = usePosts() // Add a post addPost(newPost) return { posts } } } ```
Conclusion
Choosing the right state management tool depends on the specifics of your project, a decision that becomes less daunting when you hire Vue.js developers. Vuex, with its comprehensive solution, is a great fit for complex applications, and Vue.js developers are well versed in using it. However, the added complexity and boilerplate might be overkill for smaller ones. Alternatives like Vue.Observable and Pinia provide a more straightforward approach, whereas Vue Apollo is an excellent choice if your application relies heavily on a GraphQL API. Finally, the Vue Composition API, in combination with Vue’s reactivity system, can provide an efficient and flexible way to manage state.
State management is a complex topic and requires thoughtful decisions. This is why many organizations choose to hire Vue.js developers. It’s always a good practice to evaluate the needs of your project before choosing a tool. The examples in this blog post should serve as a starting point for understanding how these tools can be used to manage state in a Vue.js application, whether you’re a developer looking to expand your skills, or a business looking to hire Vue.js developers. Happy coding!
Table of Contents
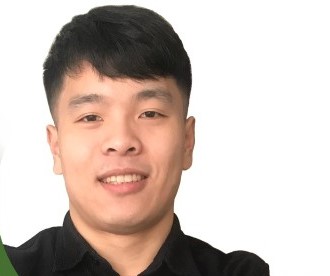
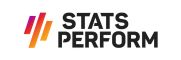