Vue.js Testing Strategies: Ensuring Quality in Your Applications
In the ever-evolving world of web development, Vue.js has emerged as one of the most popular and versatile JavaScript frameworks. Its simplicity, scalability, and ease of use have attracted developers across the globe. However, with the increasing complexity of web applications, ensuring their quality has become a crucial aspect of the development process. That’s where Vue.js testing strategies come into play.
Table of Contents
Testing is an integral part of software development, allowing developers to identify and fix bugs, prevent regressions, and build reliable applications. In this blog post, we’ll explore the essential testing strategies for Vue.js applications, ranging from unit testing to component testing and end-to-end testing. By the end of this guide, you’ll be equipped with the knowledge to write tests that guarantee the robustness and stability of your Vue.js applications.
1. Why Test Vue.js Applications?
Testing Vue.js applications brings several benefits to the development process. Here are some key reasons why testing is essential:
1.1. Bug Detection and Prevention
Tests help you identify bugs early in the development cycle, making it easier to fix them before they reach production. By continuously running tests, you prevent regressions and ensure that new features don’t break existing functionality.
1.2. Improved Code Quality
Writing tests encourages you to write modular and maintainable code. It allows you to design your application with testability in mind, leading to cleaner and more organized code.
1.3. Faster Development and Deployment
While writing tests may seem time-consuming initially, it saves a lot of time in the long run. Catching bugs early means fewer issues to fix later. Moreover, automated testing allows you to streamline your development process and deploy with confidence.
1.4. Better Collaboration
Testing enhances collaboration between team members. Developers can understand the requirements better by looking at the tests, and QA teams can ensure that the application meets the desired specifications.
Now, let’s dive into the various testing strategies for Vue.js applications.
2. Unit Testing
Unit testing is the foundation of testing Vue.js applications. It involves testing individual units of code in isolation, usually functions or methods, to ensure they work as expected. By testing units independently, you can easily identify where a bug or failure occurs.
2.1. Writing a Unit Test
Let’s consider a simple Vue.js component, the Counter, which increments a value on each button click.
vue <template> <div> <p>{{ count }}</p> <button @click="increment">Increment</button> </div> </template> <script> export default { data() { return { count: 0, }; }, methods: { increment() { this.count++; }, }, }; </script>
To write a unit test for the increment method, you can use testing libraries like Jest or Mocha with Vue Test Utils.
js import { shallowMount } from '@vue/test-utils'; import Counter from '@/components/Counter.vue'; describe('Counter', () => { it('increments the count when the button is clicked', () => { const wrapper = shallowMount(Counter); const button = wrapper.find('button'); button.trigger('click'); expect(wrapper.vm.count).toBe(1); }); });
In this example, we shallow-mount the Counter component and simulate a button click to test if the count increments as expected.
3. Component Testing
Unit tests are great for testing isolated pieces of logic, but they might not be sufficient to ensure the smooth integration of components within a Vue.js application. Component testing focuses on verifying how different components interact with each other and how they render when used together.
3.1. Writing a Component Test
Consider a simple Vue.js application with two components, Parent and Child, where the Child component is nested within the Parent.
vue <template> <div> <h1>{{ title }}</h1> <Child :name="childName" /> </div> </template> <script> import Child from './Child.vue'; export default { components: { Child, }, data() { return { title: 'Testing Vue.js', childName: 'Alice', }; }, }; </script>
To test the interaction between the Parent and Child components, we can use Vue Test Utils to shallow-mount the Parent and verify its behavior.
js import { shallowMount } from '@vue/test-utils'; import Parent from '@/components/Parent.vue'; import Child from '@/components/Child.vue'; describe('Parent', () => { it('renders the title and passes the correct props to Child', () => { const wrapper = shallowMount(Parent); const title = wrapper.find('h1'); const childComponent = wrapper.findComponent(Child); expect(title.text()).toBe('Testing Vue.js'); expect(childComponent.props('name')).toBe('Alice'); }); });
In this example, we test the rendering of the title in the Parent component and verify that the correct props are being passed to the Child component.
4. End-to-End Testing
While unit and component testing are essential, they might not catch all issues that arise from the actual user interactions and flow of the application. End-to-end (E2E) testing verifies the complete application workflow from the user’s perspective.
4.1. Writing an End-to-End Test
To perform E2E testing in Vue.js applications, we can use tools like Cypress, which provides an elegant and straightforward API to interact with the application.
Suppose we have a simple form component that takes user input and displays a message based on the input.
vue <template> <div> <input v-model="name" type="text" /> <button @click="submit">Submit</button> <p v-if="submitted">Hello, {{ name }}!</p> </div> </template> <script> export default { data() { return { name: '', submitted: false, }; }, methods: { submit() { this.submitted = true; }, }, }; </script>
To write an E2E test using Cypress, we need to create a test file and use the Cypress API to interact with the application.
js // cypress/integration/form.spec.js describe('Form', () => { it('submits the form and displays a greeting message', () => { cy.visit('/'); // Assuming the application is running at localhost:8080 cy.get('input').type('John Doe'); cy.get('button').click(); cy.contains('Hello, John Doe!').should('be.visible'); }); });
In this test, we visit the application, type a name in the input field, click the submit button, and then verify that the greeting message is displayed.
Conclusion
Vue.js testing strategies play a vital role in ensuring the quality and reliability of your applications. By leveraging unit testing, component testing, and end-to-end testing, you can confidently build and deploy robust Vue.js applications. Automated tests not only catch bugs early in the development cycle but also enhance collaboration among team members and facilitate faster development and deployment.
Remember, testing is an ongoing process, and as your application grows, so will your test suite. Embrace testing as an integral part of your Vue.js development journey, and you’ll reap the benefits of high-quality, maintainable, and resilient applications. Happy testing!
Table of Contents
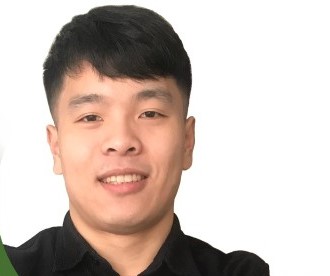
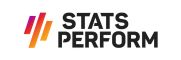