Working with APIs in Vue.js: Consuming Data from External Sources
In the modern web development landscape, building dynamic and data-driven applications has become the norm. One of the most crucial aspects of creating such applications is working with Application Programming Interfaces (APIs). APIs allow developers to access and consume data from external sources, providing real-time information and enriching the user experience. Vue.js, with its reactivity and ease of use, is an excellent choice for building interactive front-end applications that can consume data from APIs seamlessly.
Table of Contents
In this blog post, we will explore how to work with APIs in Vue.js, covering the steps required to fetch and display data from external sources. We’ll delve into some essential concepts and demonstrate how to incorporate APIs into your Vue.js projects effectively.
1. Prerequisites
Before we begin, ensure you have the following prerequisites:
2. Basic knowledge of Vue.js.
A code editor (e.g., Visual Studio Code).
Node.js and npm (Node Package Manager) installed on your system.
3. Understanding APIs
3.1. What is an API?
An API, or Application Programming Interface, is a set of rules and protocols that allows different software applications to communicate with each other. It defines the methods and data formats that applications can use to request and exchange information. APIs enable developers to access external services, retrieve data, and perform various operations over the internet.
3.2. Types of APIs
- RESTful APIs: Representational State Transfer (REST) APIs are one of the most common types used in web development. They utilize standard HTTP methods (GET, POST, PUT, DELETE) to interact with resources identified by URLs. Responses are usually in JSON or XML format.
- GraphQL APIs: GraphQL is a query language that allows clients to request specific data from the server. Unlike REST APIs, where each endpoint returns a fixed set of data, GraphQL provides more flexibility, as clients can specify the exact data they need.
- Third-party APIs: These are APIs provided by external services, allowing developers to access features or data from those services. Examples include social media APIs, weather APIs, and payment gateway APIs.
4. Setting Up a Vue.js Project
Before we dive into consuming APIs, let’s set up a new Vue.js project to work with. We’ll use Vue CLI (Command Line Interface) for this purpose.
Step 1: Install Vue CLI
If you haven’t installed Vue CLI globally, open your terminal and run the following command:
bash npm install -g @vue/cli
Step 2: Create a New Vue Project
Once Vue CLI is installed, create a new Vue.js project using the following command:
bash vue create my-api-project
Replace “my-api-project” with the desired project name. The Vue CLI will prompt you to select preset configurations; you can either choose the default or manually select features according to your needs.
Step 3: Navigate to the Project Directory
Move into the newly created project directory:
bash cd my-api-project
Step 4: Serve the Vue Application
To verify that your project is set up correctly, serve the Vue application:
bash npm run serve
Visit http://localhost:8080 in your browser; you should see the Vue.js welcome page.
Now that our Vue.js project is ready, let’s proceed to consume data from external APIs.
5. Making API Requests in Vue.js
In Vue.js, we can use various methods to make API requests. We’ll cover two common approaches: using the axios library and utilizing the built-in fetch API.
Method 1: Using Axios
Axios is a popular JavaScript library used for making HTTP requests. It offers a straightforward and flexible API for handling API calls.
Step 1: Install Axios
Install Axios in your project by running the following command:
bash npm install axios
Step 2: Import Axios
In your Vue component where you want to make the API request, import Axios:
javascript import axios from 'axios';
Step 3: Make the API Request
Now, let’s make a GET request to a sample API endpoint and display the data.
javascript <template> <div> <h1>Data from API:</h1> <ul> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> </div> </template> <script> export default { data() { return { items: [] }; }, mounted() { axios .get('https://api.example.com/data') .then(response => { this.items = response.data; }) .catch(error => { console.error('Error fetching data:', error); }); } }; </script>
In this example, we use the axios.get() method to make a GET request to the https://api.example.com/data endpoint. When the response is received successfully, we update the items array in the component’s data with the retrieved data, which will automatically reflect in the template.
Method 2: Using Fetch API
Vue.js also supports the native fetch API for making network requests, which is supported by modern browsers.
Step 1: Make the API Request
javascript <template> <div> <h1>Data from API:</h1> <ul> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> </div> </template> <script> export default { data() { return { items: [] }; }, mounted() { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { this.items = data; }) .catch(error => { console.error('Error fetching data:', error); }); } }; </script>
In this approach, we use the fetch() method to make a GET request to the API endpoint. The response is then converted to JSON using the .json() method, and the data is set to the items array in the component.
Both axios and fetch are effective ways to handle API requests in Vue.js. Choose the one that best fits your project’s requirements and team preferences.
6. Handling API Errors
Error handling is a crucial aspect when working with APIs. In the previous examples, we already included simple error handling using .catch() to display an error message in the console. However, for a better user experience, you should implement more robust error handling and display error messages on your application’s interface.
Example: Enhanced Error Handling
javascript <template> <div> <h1>Data from API:</h1> <ul v-if="!error"> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> <p v-else>{{ error }}</p> </div> </template> <script> export default { data() { return { items: [], error: null }; }, mounted() { fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => { this.items = data; }) .catch(error => { this.error = 'Error fetching data: ' + error.message; console.error(this.error); }); } }; </script>
In this enhanced example, we have added a conditional rendering to display either the data from the API or the error message based on the value of the error property in the component’s data. If the response status is not okay (i.e., not in the 2xx range), we throw an error and handle it in the .catch() block.
This provides a more user-friendly experience, allowing your users to understand when there’s a problem with the API request.
7. Incorporating API Data in Components
After fetching data from an API, you can integrate it into your Vue components to display and interact with the information effectively.
7.1. Rendering API Data
Continuing from the previous examples, let’s create a component that renders the API data we fetched.
Step 1: Create a new component
Create a new Vue component, ApiData.vue, using the following command:
bash vue create src/components/ApiData.vue
Step 2: Modify the component
Now, open ApiData.vue and modify it as follows:
javascript <template> <div> <h1>Data from API:</h1> <ul> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> </div> </template> <script> export default { props: { items: { type: Array, required: true } } }; </script>
In this component, we define a prop called items, which will receive the API data array. The component will then render this data using the same code as in the previous examples.
Step 3: Use the component in App.vue
Now that we have the ApiData.vue component, let’s use it in the App.vue component:
javascript <template> <div> <h1>My Vue.js API Project</h1> <ApiData :items="items" /> </div> </template> <script> import ApiData from './components/ApiData.vue'; export default { components: { ApiData }, data() { return { items: [] }; }, mounted() { // Make API request and update 'items' data as shown in previous examples } }; </script>
Here, we import the ApiData.vue component and register it in the components option of App.vue. We then pass the items array as a prop to the ApiData component, which will handle rendering the data.
By breaking down your application into smaller components, you ensure a cleaner and more maintainable codebase.
8. Implementing Data Manipulation with APIs
Apart from fetching data, APIs also allow us to perform other operations like creating, updating, and deleting data. Let’s look at how to implement these operations in Vue.js.
Example: Creating Data via API
In this example, we’ll demonstrate how to create new data using a POST request to the API endpoint.
javascript <template> <div> <h1>Create a New Item:</h1> <form @submit.prevent="createItem"> <input type="text" v-model="newItemName" /> <button type="submit">Add Item</button> </form> <ul> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> </div> </template> <script> export default { data() { return { items: [], newItemName: '' }; }, mounted() { // Fetch existing items as shown in previous examples }, methods: { createItem() { // Send a POST request to the API with the newItemName fetch('https://api.example.com/data', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ name: this.newItemName }) }) .then(response => response.json()) .then(data => { this.items.push(data); this.newItemName = ''; }) .catch(error => { console.error('Error creating item:', error); }); } } }; </script>
In this example, we’ve added a form with an input field to capture the name of the new item. When the user submits the form, the createItem method is called. Inside this method, we make a POST request to the API endpoint with the new item’s name as the request payload.
Upon receiving a successful response, we add the newly created item to the items array and clear the input field for the next item to be added.
Conclusion
In this blog post, we have explored how to work with APIs in Vue.js to consume data from external sources. We covered different types of APIs, set up a Vue.js project, and demonstrated how to make API requests using Axios and the fetch API. We also discussed handling API errors and integrating API data into Vue components. Additionally, we implemented data manipulation operations such as creating new data via POST requests.
Working with APIs in Vue.js opens up a world of possibilities for creating dynamic and interactive web applications that can leverage real-time data and external services. The knowledge gained from this blog will empower you to build feature-rich applications that enrich the user experience and keep your users engaged.
Now it’s time to put your new skills to practice and start building amazing Vue.js applications powered by APIs! Happy coding!
Table of Contents
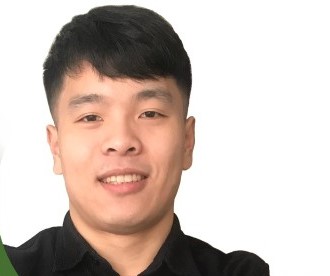
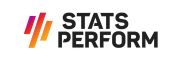